Day 02: Process States and Transitions - A Comprehensive Exploration
Table of Contents
- Introduction
- Fundamental Process States
- State Transition Mechanisms
- State Transition Algorithms
- Practical Implementation
- Code Examples
- Compilation and Execution Instructions
- References and Further Reading
- Conclusion
1. Introduction
Process states represent the dynamic lifecycle of computational execution. Understanding these states and their transitions is crucial for grasping how operating systems manage computational resources efficiently.
2. Fundamental Process States
a. New State
- Process is being created
- Resources are being allocated
- Not yet ready for execution
- Initialization of process control block (PCB)
b. Ready State
- Process is prepared for execution
- Waiting in the process queue
- All required resources are available
- Awaiting CPU scheduler selection
c. Running State
- Process is currently executing
- Actively using CPU resources
- Instructions are being processed
- Can transition to other states based on events
d. Waiting (Blocked) State
- Process is temporarily suspended
- Waiting for external event
- Common triggers:
- I/O operations
- Inter-process communication
- Resource allocation
- External synchronization signals
e. Terminated State
- Process execution is complete
- Resources are being deallocated
- Process control block is being removed
- Memory is being freed
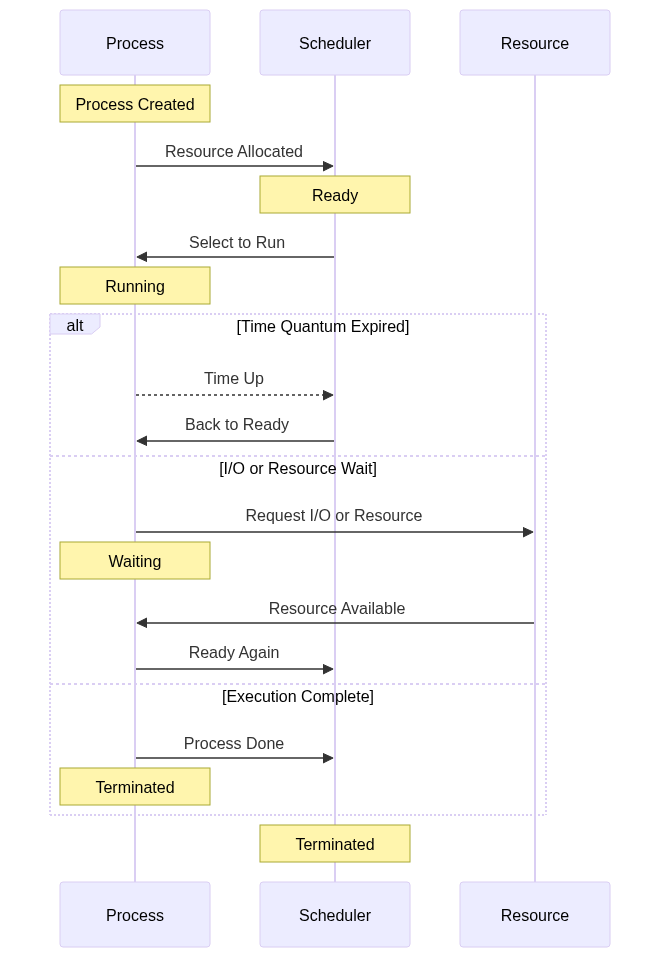
3. State Transition Mechanisms
Transition Types
- Scheduler-Driven Transitions
- Moving between Ready and Running states
- Controlled by CPU scheduling algorithms
- Event-Driven Transitions
- Moving to Waiting state due to external events
- Triggered by I/O, resource constraints
- Interrupt-Based Transitions
- Sudden state changes due to hardware or software interrupts
- Ensures system responsiveness and multitasking
4. State Transition Algorithms
Round-Robin Scheduling
- Circular queue of processes
- Fixed time quantum for each process
- Ensures fair CPU time distribution
Priority-Based Transitions
- Processes assigned priority levels
- Higher priority processes get preferential state transitions
- Prevents starvation of low-priority processes
5. Practical Implementation Considerations
Context Switching
- Saving and restoring process states
- Minimal overhead during transitions
- Preserving process execution context
Race Conditions
- Synchronization challenges during state transitions
- Need for atomic state change operations
- Mutex and semaphore mechanisms
6. Code Example: Process State Simulation
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <pthread.h>
// Process state enumeration
typedef enum {
NEW,
READY,
RUNNING,
WAITING,
TERMINATED
} ProcessState;
// Process Control Block (Simplified)
typedef struct {
int pid;
ProcessState state;
int priority;
} PCB;
// Function to simulate state transition
void transition_state(PCB *process, ProcessState new_state) {
printf("Transitioning Process %d: %d -> %d\n",
process->pid, process->state, new_state);
process->state = new_state;
}
int main() {
PCB process;
process.pid = getpid();
process.state = NEW;
process.priority = 5;
// Simulating state transitions
transition_state(&process, READY);
sleep(1); // Simulate preparation time
transition_state(&process, RUNNING);
sleep(2); // Simulate execution
transition_state(&process, WAITING);
sleep(1); // Simulate waiting for resource
transition_state(&process, READY);
sleep(1);
transition_state(&process, TERMINATED);
return 0;
}
7. Compilation and Execution Instructions
For Linux/Unix Systems:
# Compile the program
gcc -o process_states process_states.c -pthread
# Run the executable
./process_states
Compilation Flags Explanation
-o process_states
: Specify output executable name
-pthread
: Link with POSIX threads library
- Ensures proper compilation with thread support
8. References and Further Reading
Academic References
- Silberschatz, A., Galvin, P. B., & Gagne, G. (2018). Operating System Concepts
- Tanenbaum, A. S. (2006). Modern Operating Systems
Online Resources
- Documentation
- Linux Process Management Docs: https://www.kernel.org/doc/html/latest/process/index.html
- POSIX Thread Programming: https://pubs.opengroup.org/onlinepubs/9699919799/
- Stack Exchange Discussions
- Unix & Linux Stack Exchange - Process Management: https://unix.stackexchange.com/questions/tagged/process
Video Tutorials
- MIT OpenCourseWare: Operating Systems Lectures
- Berkeley CS162 Operating Systems Course Videos
9. Conclusion
Process states and transitions form the core of computational resource management. By understanding these mechanisms, developers and system administrators can optimize system performance, manage resources efficiently, and design robust multitasking environments.
Key Insights
- Processes have well-defined state lifecycles
- State transitions are governed by scheduling algorithms
- Proper state management ensures system responsiveness
Prepare for Day 3: Exploring Process Creation Mechanisms
Embrace the complexity, one state at a time!